Create a service a timer
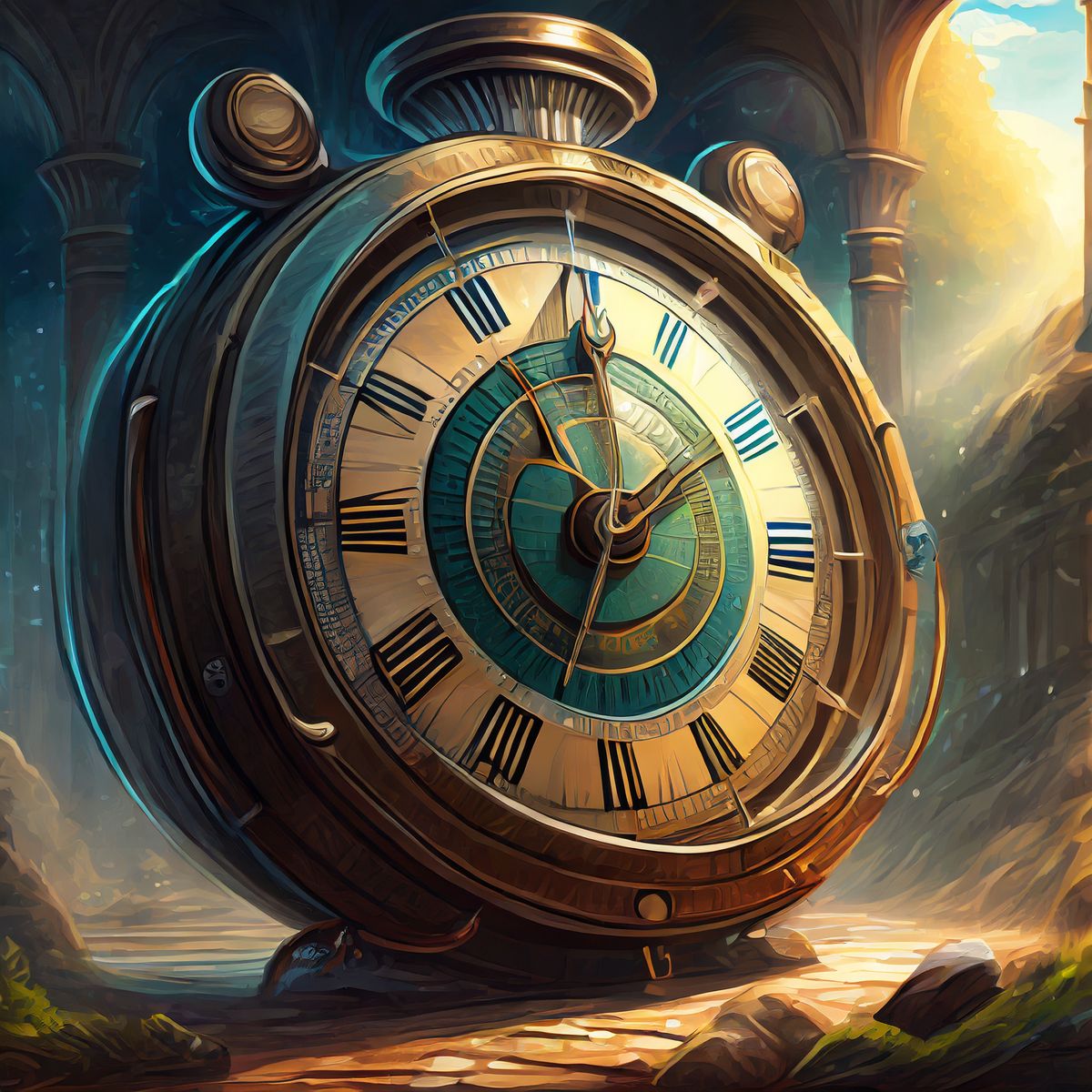
The goal here is to run a script that will select a random image and show it in the PIMORONI Inky Impression 7 Color ePaper every determined period of time. I assume python3 is already installed in the machine. The Pimorony Inky Impression libraries and examples can be downloaded from this website. I am using a Raspberry PI Zero WH with Raspbian GNU/Linux 11 (bullseye).
- Install Pimoroni's Inky libraries (you may say YES to all).
curl https://get.pimoroni.com/inky | bash
- Go inside the directory where services are.
cd /etc/systemd/system/
- Create a the timer file.
Add the following content to it:nano inky7.timer
Save and exit.[Unit] Description=PIMORONI Inky Impression 7 color e-paper random image select and display TIMER. [Timer] OnUnitActiveSec=180s OnBootSec=180s [Install] WantedBy=timers.target
- Create a the service file.
Add the following content to it:nano inky7.service
Save and exit.[Unit] Description=Description=PIMORONI Inky Impression 7 color e-paper random image select and display JOB. [Service] Type=oneshot SyslogIdentifier=inky7_service ExecStart=bash /usr/local/bin/inky7.sh
- Create the script file.
Add the following content to it:nano /usr/local/bin/inky7.sh
Save and exit.ls /home/pi/Pictures/Inky/*.jpg | sort -R | tail -1 | while read file; do python3 /usr/local/bin/show.py $file; done
- Create the Python file.
Add the following content to it (careful with the indentation):nano /usr/local/bin/show.py
#!/usr/bin/env python3 # File Name: show.py # # Usage 1: Show a selected image # # python3 show.py foto1.jpg # python3 show.py /home/pi/foto1.jpg # # Usage 2: Show a random image # # ls /home/pi/*.jpg | sort -R | tail -1 | while read file; do python3 show.py $file; done import time import sys from inky.inky_uc8159 import Inky, CLEAN from PIL import Image from inky.inky_uc8159 import Inky inky = Inky() saturation = 0.6 # Clear display for _ in range(2): for y in range(inky.height - 1): for x in range(inky.width - 1): inky.set_pixel(x, y, CLEAN) inky.show() time.sleep(1.0) # Load image if len(sys.argv) == 1: print(""" Usage: {file} image-file """.format(file=sys.argv[0])) sys.exit(1) image = Image.open(sys.argv[1]) if len(sys.argv) > 2: saturation = float(sys.argv[2]) inky.set_image(image, saturation=saturation) inky.show()
- Reload the daemon to take the changes into account.
systemctl daemon-reload
- Start the timer, this will start the service automatically.
systemctl start inky7.timer
- Enable the timer so that it runs at startup, this is optional.
systemctl enable inky7.timer
Example logs (journalctl):
Jan 23 20:38:49 raspberrypi systemd[1]: Starting Description=PIMORONI Inky Impression 7 color e-paper random image select and display JOB....
Jan 23 20:39:49 raspberrypi systemd[1]: inky7.service: Succeeded.
Jan 23 20:39:49 raspberrypi systemd[1]: Finished Description=PIMORONI Inky Impression 7 color e-paper random image select and display JOB..
Jan 23 20:39:49 raspberrypi systemd[1]: inky7.service: Consumed 22.214s CPU time.
Jan 23 20:42:49 raspberrypi systemd[1]: Starting Description=PIMORONI Inky Impression 7 color e-paper random image select and display JOB....
Jan 23 20:43:49 raspberrypi systemd[1]: inky7.service: Succeeded.
Jan 23 20:43:49 raspberrypi systemd[1]: Finished Description=PIMORONI Inky Impression 7 color e-paper random image select and display JOB..
Jan 23 20:43:49 raspberrypi systemd[1]: inky7.service: Consumed 22.266s CPU time.
Notes:
To stop the timer and the service respectively run:
systemctl stop inky7.timer
systemctl stop inky7.service
To stop running at startup run:
systemctl disable inky7.timer