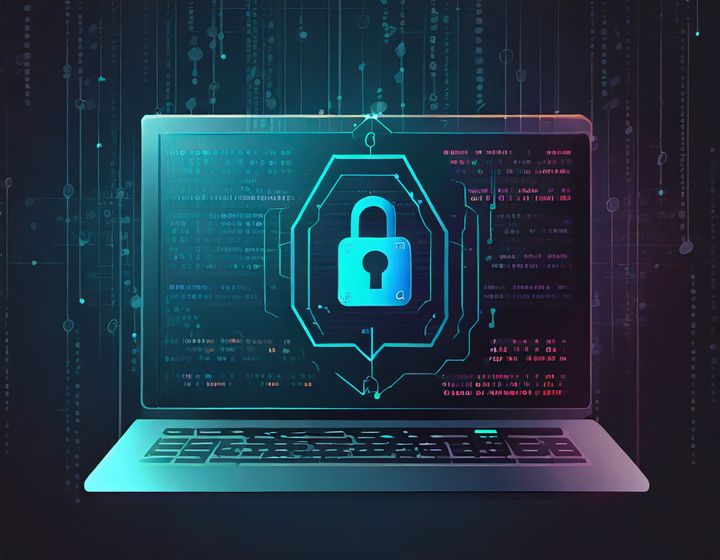
Copy-Paste-Run-Ready string encryption / decryption with AES-256 in Node.JS
/* --- Code ready to copy -> paste -> run --- */
// Libraries
const crypto = require('crypto');
const ENCODING = "hex"; // hex or base64
// Implementations
function encryptAES256(clearMsg, key) {
let iv = crypto.randomBytes(16); // Generating 16 byte random IV
let cipher = crypto.createCipheriv(
"aes256",
crypto.createHash("sha256").update(key, "utf8").digest(), // 32 bytes