Shortest and simplest string encryption / decryption with AES-256 in Node.JS
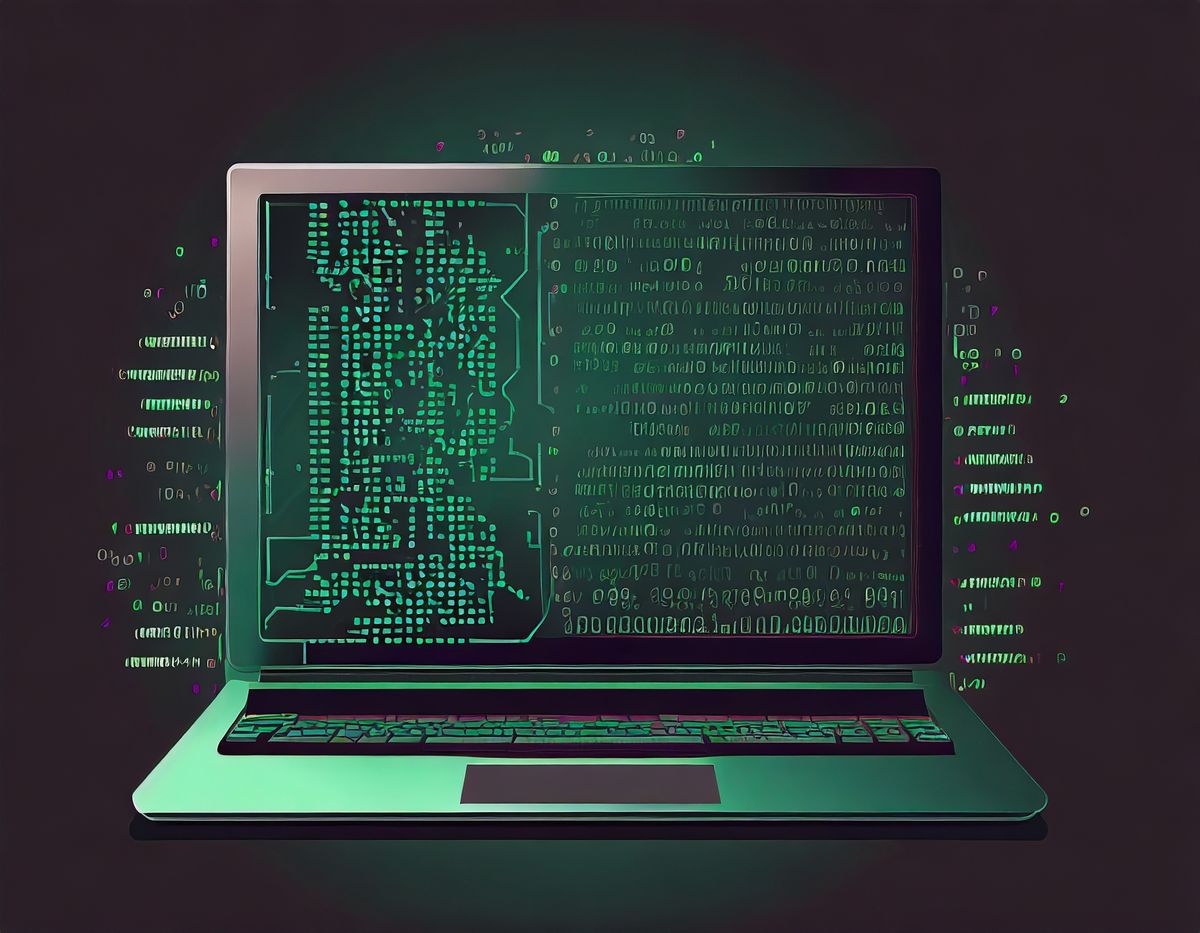
Copy and paste the following code in a .js file, then run it.
// Libraries
const crypto = require('crypto');
// Constants
const OUTPUT_FORMAT = "hex";
const ALGORITHM = "aes256";
const KEY = "012345ABCDEFGHIJKLMNOPQRSTUVWXYZ";
const IV = "ABCDEF0123456789";
// Implementations
function encrypt(message) {
let cipher = crypto.createCipheriv(
ALGORITHM,
Buffer.from(KEY),
Buffer.from(IV)
);
return (Buffer.concat([
cipher.update(message),
cipher.final()
])).toString(OUTPUT_FORMAT);
}
function decrypt(message) {
let decipher = crypto.createDecipheriv(
ALGORITHM,
Buffer.from(KEY),
Buffer.from(IV)
);
return (Buffer.concat([
decipher.update(Buffer.from(message, OUTPUT_FORMAT)),
decipher.final()
])).toString('utf8');
}
// Program
console.log(encrypt("Hello"));
console.log(decrypt(encrypt("Hello")));
Sample output:
c5c79e5679cb7e3fef8412c2564f8427
Hello