Password Based Key Derivation Function 2 (PBKDF2) demo in Node.JS v15
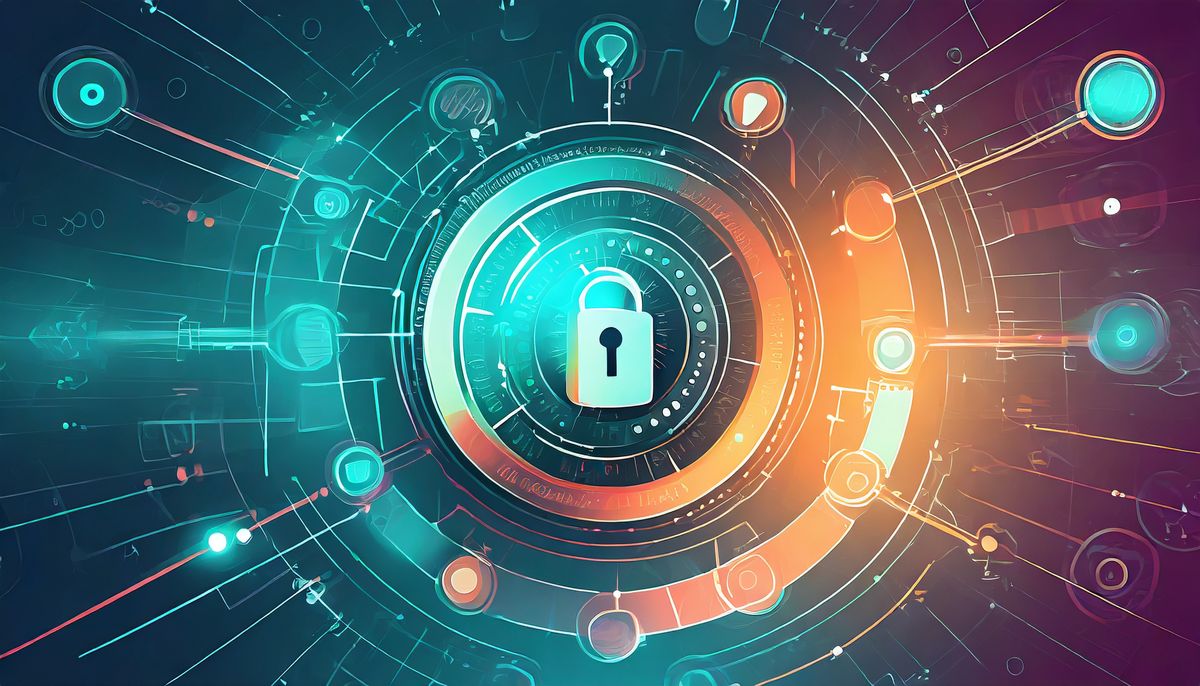
A quick demo on how to use Password Based Key Derivation Function 2 (PBKDF2) to generate keys and IVs to use with all different ciphers available in Node.JS v15.x.
const crypto = require('crypto');
const PASSWORD = "My_Secret_Password"; // Could be the password/key used to encrypt a message
const ITERATIONS = 10;
const DIGEST = "sha256";
crypto.getCiphers().forEach(cipher => {
let cipherInfo = crypto.getCipherInfo(cipher);
let keyLength = cipherInfo.keyLength;node
let ivLength = cipherInfo.ivLength;
let key = keyLength ? crypto.pbkdf2Sync(PASSWORD, cipher, ITERATIONS, keyLength, DIGEST) : "";
let iv = ivLength ? crypto.pbkdf2Sync(cipher, PASSWORD, ITERATIONS, ivLength, DIGEST) : ""; // NaN = undefined = "" for some algorithms
console.log("Cipher: %s | Key Length: %d | IV Length: %d | Key: %s | IV: %s", cipher, keyLength, ivLength, key.toString('hex'), iv.toString('hex'));
});
Your console output should be similar to this:
Cipher: aes-128-cbc | Key Length: 16 | IV Length: 16 | Key: 907b8647d46b17120f66a625021dd3c9 | IV: 3e442669285c9014c51914671e5701fd
Cipher: aes-128-cbc-hmac-sha1 | Key Length: 16 | IV Length: 16 | Key: 8f64a00aca85234eb97cfadc2d84e5b6 | IV: 84d053309610e7a716cbf817a0d85b88
Cipher: aes-128-cbc-hmac-sha256 | Key Length: 16 | IV Length: 16 | Key: 97a3e54f243b8c8f4813ea96ecf1b216 | IV: 110e2b48cd6cadca437a9a2a2004e6a2
Cipher: aes-128-ccm | Key Length: 16 | IV Length: 12 | Key: 27ed4b2536ae45ba33090e941b98c33e | IV: 1d8d7fc4c4727219aa005a87
Cipher: aes-128-cfb | Key Length: 16 | IV Length: 16 | Key: 2dda366e2ffcb5d91326b5def4723bdb | IV: 620c6797676ee4f51dac4f66c0e2af79
Cipher: aes-128-cfb1 | Key Length: 16 | IV Length: 16 | Key: f3519331e72b5cb54d095f4bd36a92f2 | IV: b20217303fee80b6e76656aa8cca9a85
Cipher: aes-128-cfb8 | Key Length: 16 | IV Length: 16 | Key: b9c33d12d4b3aff73f992c92c280b0e0 | IV: 4eb30f523c247217988a2d8fe58e56e7
Cipher: aes-128-ctr | Key Length: 16 | IV Length: 16 | Key: f5b245e5f279fd9c9b157191c677036c | IV: e5b55a588100d9fda9f83e041a15a146
Cipher: aes-128-ecb | Key Length: 16 | IV Length: NaN | Key: 7ee428db46a1e5ea4f56157e61ece823 | IV:
Cipher: aes-128-gcm | Key Length: 16 | IV Length: 12 | Key: 38594d31ab81e5544f0b7a7921c1ae70 | IV: e67ed442a7ab5a47ed8313fa
Cipher: aes-128-ocb | Key Length: 16 | IV Length: 12 | Key: 91def5ad6fb05ac4f5e4d21542520853 | IV: e309acee26c37980a0179910
Cipher: aes-128-ofb | Key Length: 16 | IV Length: 16 | Key: cdaad1464b3179b07315949129200aff | IV: fad81c8a95e4c149e23b09bae324592a
Cipher: aes-128-xts | Key Length: 32 | IV Length: 16 | Key: c8e953ed33723bafde1f2256824bc5086b2fb7b431e31a16389309e32d15b8a2 | IV: e326064d9ed8bfbeb1347251674cc287
Cipher: aes-192-cbc | Key Length: 24 | IV Length: 16 | Key: def1223fd5baf28bae564e355afcd6612ca80c49ca155360 | IV: 45ada542361a6542f88599fded1d92ad
Cipher: aes-192-ccm | Key Length: 24 | IV Length: 12 | Key: b58c5f83802a1f65f32eadf9a7b3ac7bfd5362e20b400b26 | IV: 1868e22f5c3869029fb63fa5
Cipher: aes-192-cfb | Key Length: 24 | IV Length: 16 | Key: 2ade7de321f4d3a8775beaa89546ece33f2e94c68f9a5478 | IV: 54ab97cc16a41f1ad415d8bd2ce04631
Cipher: aes-192-cfb1 | Key Length: 24 | IV Length: 16 | Key: 1de0bd90cb0ba66ab9de40300dfdf45cfb88fef11258a1ee | IV: 29a5e040f0176ce6ce6c243deb6b8375
Cipher: aes-192-cfb8 | Key Length: 24 | IV Length: 16 | Key: b6bea58db913668d37d8f3080a03f7a91b354398e55076a5 | IV: 39a511b041fbbedeb1c5a489521143b3
Cipher: aes-192-ctr | Key Length: 24 | IV Length: 16 | Key: 8dd418674d5dc409288bf93a83c104ae89cad25f20617dab | IV: e91a5e5a8c8d57d55b3287809776b456
Cipher: aes-192-ecb | Key Length: 24 | IV Length: NaN | Key: 4bb90cb6e19a5ddd24b9321e7d030109d98b2109f6b0a583 | IV:
Cipher: aes-192-gcm | Key Length: 24 | IV Length: 12 | Key: 8ff793eab5b4fc73bb324440ad1a03275bb751877ddc3919 | IV: 3770270f67298b6ad5dca280
Cipher: aes-192-ocb | Key Length: 24 | IV Length: 12 | Key: f68a2be4525742331f0c4fa7b72b65b7f3db783a2a8b2ad3 | IV: 45bbc44a7abc980f6c375fb1
Cipher: aes-192-ofb | Key Length: 24 | IV Length: 16 | Key: 2c2dd0ae1d9e14a2bba239e9babdc71b1441681a7bc94ffb | IV: 3c5021c51b55e3b6f9439649f98f5d2b
Cipher: aes-256-cbc | Key Length: 32 | IV Length: 16 | Key: 7f74b1a95bd6a4ccbfcb6475faa799e117b693fe0cd945b5149d4b31435c31e2 | IV: 1432984dcd7414a9ab69ac482bac3a8a
Cipher: aes-256-cbc-hmac-sha1 | Key Length: 32 | IV Length: 16 | Key: c59815983d6d40e63f67e34f2240f9eb4bb727464c75213bae1f53363f5b8ee1 | IV: 27f7ceed85c05ce5fb6b9f288cff2198
Cipher: aes-256-cbc-hmac-sha256 | Key Length: 32 | IV Length: 16 | Key: f23519d4a4db97fa538befe4087f29108513ffb7e57d6bf45c413e28ce856e51 | IV: 3899a41542b56b9f4544b5c1acd7baa9
Cipher: aes-256-ccm | Key Length: 32 | IV Length: 12 | Key: 05dbc63c7d7744ef93c6e39b89cbdfbd40cdffbd1099873f6f27f9d18541b4b1 | IV: 32fee10b277837c731f6b42a
Cipher: aes-256-cfb | Key Length: 32 | IV Length: 16 | Key: 7120081dea4dc52e2d7653ea57849a76c8e0e06e9ba8fe6487b00a33b413c674 | IV: 34e06329344f83bab413bf74623ca34e
Cipher: aes-256-cfb1 | Key Length: 32 | IV Length: 16 | Key: be6a929bf2ef4b061e8e4214cb6a3172ba464eaa2cbad7e0ab335add8be76c2b | IV: 790b37e7ec2e6bf51d38e205104b1840
Cipher: aes-256-cfb8 | Key Length: 32 | IV Length: 16 | Key: 37e1a83e509bdf732171111cd204dcb65623571f34f5be926e0aa88a0ab59f32 | IV: b58e158279f46b7832cfc700750fb69c
Cipher: aes-256-ctr | Key Length: 32 | IV Length: 16 | Key: ee35553727804eb80061f392aba470541ca7f5257ab89213777d568bad51c0e8 | IV: f7e82555c791bb4a01e382d76d4ed1fa
Cipher: aes-256-ecb | Key Length: 32 | IV Length: NaN | Key: 4002b6a286e68cdeef154fc93a77ebec90442f4b33fe00e8655eacd3de6858ee | IV:
Cipher: aes-256-gcm | Key Length: 32 | IV Length: 12 | Key: 575c5cfbba8cd19d7d861706d348acd9acf1cc499bd8c12fe18a246e00a4e519 | IV: f2614f43d7be9c6da4bf947e
Cipher: aes-256-ocb | Key Length: 32 | IV Length: 12 | Key: 3772454f4b422b3ff8cb89fb0fc61b06d0eac3b382c44b01156dfceb73691609 | IV: 10764764edd4369d95d40cb4
Cipher: aes-256-ofb | Key Length: 32 | IV Length: 16 | Key: 9f4361688457e12b8fc1c01afca19c72eadceac1551c7df45820ddbd2c17b565 | IV: 2f5d89f4fbd44a3cadbc98c8d72811eb
Cipher: aes-256-xts | Key Length: 64 | IV Length: 16 | Key: 0c0cd297119266c161a4762b8779cf7c230be9eb16c1d2f3ea8ceb595f164efd83b195aa126282bd68239eaf6dc0280911c43e48fe74f7f8e2a3cde6779543cb | IV: e94731efd455edbe70040aa4d7d6cfa2
Cipher: aes128 | Key Length: 16 | IV Length: 16 | Key: d9543bc760f46135fffd6796e1c1bbd4 | IV: 1178d25f6f669fff90dac0715c1d295f
Cipher: aes128-wrap | Key Length: 16 | IV Length: 8 | Key: a2d331b5cd0404d1d600e478c79b7dc4 | IV: 892babe20c1bc2ab
Cipher: aes192 | Key Length: 24 | IV Length: 16 | Key: dbb5ad155ac325198e31695f6f80eee241e4e54017c98e0e | IV: d8bbd3cbff140b67ee9beced4a254a92
Cipher: aes192-wrap | Key Length: 24 | IV Length: 8 | Key: a818b82767957f6862aab824ae4f9213a0c2770cf2e6433d | IV: 18f10383e061b432
Cipher: aes256 | Key Length: 32 | IV Length: 16 | Key: dcc539e894e386ed32c10b4f2285d8c9f38c2b4453dd5353cf10563df3f5b28c | IV: 8710494ec4e1a017b707d848cd1abb8b
Cipher: aes256-wrap | Key Length: 32 | IV Length: 8 | Key: 9c2c2fd1384450cbc9ec76371a1a64f7d169708d04e2bd9153b29ea894afbcee | IV: 20156ee91455e1f7
Cipher: aria-128-cbc | Key Length: 16 | IV Length: 16 | Key: 1d904079feaf4fc682123cee4ed69ef2 | IV: 35a858932a8e838998bdcc7a67ff8645
Cipher: aria-128-ccm | Key Length: 16 | IV Length: 12 | Key: 25631f3c131e9d23de7a18b7be8af5f2 | IV: 529f78c6b9d39697da8e290e
Cipher: aria-128-cfb | Key Length: 16 | IV Length: 16 | Key: 569262f0dfa1a852777af5aa487f0388 | IV: cde417aa80c1b69abb1c86617ea77fe5
Cipher: aria-128-cfb1 | Key Length: 16 | IV Length: 16 | Key: 0344554381fdd242f81aa85074ae62f9 | IV: 90035798484fb27c561fd1b3d97242bb
Cipher: aria-128-cfb8 | Key Length: 16 | IV Length: 16 | Key: 320a7006b7b6c5bd32df170c2e3ddfe1 | IV: f071b4cb0d4ecc4740630ec7f94675d7
Cipher: aria-128-ctr | Key Length: 16 | IV Length: 16 | Key: b61c0426f1dfdf2f17fc51ef5c7317ba | IV: 5fc56e75165b4cda0e2bf28d1922894b
Cipher: aria-128-ecb | Key Length: 16 | IV Length: NaN | Key: 590fac0b2654fadee3729382d9c8708f | IV:
Cipher: aria-128-gcm | Key Length: 16 | IV Length: 12 | Key: bd21735d170eaa96bde5093d2c345277 | IV: df7b64538b03785c561afa51
Cipher: aria-128-ofb | Key Length: 16 | IV Length: 16 | Key: e4cc1b9469f9b6ea7cd4fe3c12501a4c | IV: 6125617fb6e80ad95fb65ea9ddb1aecd
Cipher: aria-192-cbc | Key Length: 24 | IV Length: 16 | Key: dae5e6bf3f641b412fa140282762689fea7ec9252f9e0364 | IV: 77c6dc020ca5ef8a1eee9479d97e670a
Cipher: aria-192-ccm | Key Length: 24 | IV Length: 12 | Key: c8f2aeabfce504b21f63b5daab88b2d49fbc5166a58e9063 | IV: af9ca7e31d8db26cd9f6e450
Cipher: aria-192-cfb | Key Length: 24 | IV Length: 16 | Key: 9feda04861634a8ce610d2337077482e75b1a581f9353522 | IV: 3596a0277c4da0423c4ec9081eb05eec
Cipher: aria-192-cfb1 | Key Length: 24 | IV Length: 16 | Key: 21c29c6b43e8e8068fa9eca3dc047bb99c572fa7559868fb | IV: ec987b3478258da1aa6cc93db1c1f9f4
Cipher: aria-192-cfb8 | Key Length: 24 | IV Length: 16 | Key: bb813e2a88fadefc930f706b0eadd73e5ef275424e1e6ffb | IV: 7c2a38e0a93a32643894066dabafacd0
Cipher: aria-192-ctr | Key Length: 24 | IV Length: 16 | Key: e489070efce6ad1ecf3975a91d8c25b7964a2681d877cfba | IV: f4c175989793dd9306a6e8cd0b20c67d
Cipher: aria-192-ecb | Key Length: 24 | IV Length: NaN | Key: a5c87bfcfcbd13c8eea7967fa8a18d0df4e57ee7588633f7 | IV:
Cipher: aria-192-gcm | Key Length: 24 | IV Length: 12 | Key: a6b589af84f8c6ce9f2466ee8fadda9163179ca4d8822ca1 | IV: 8239eee1475ebb7eca8dbd91
Cipher: aria-192-ofb | Key Length: 24 | IV Length: 16 | Key: bdde62dd58fd19a023d3be1cc680e496453186dff37970d1 | IV: 77f9502fad93511c9f3301aa91020f33
Cipher: aria-256-cbc | Key Length: 32 | IV Length: 16 | Key: aff50d764176708a8415dc78586be92e96c0860081fea1fdd6176fe4f5ade348 | IV: 7091910fc4156bbe2a91e7b5e31aaa4c
Cipher: aria-256-ccm | Key Length: 32 | IV Length: 12 | Key: f50981b0599957b6f21d593463de8a13a426375f82fce3779ff850535dd3aaa7 | IV: 6d957a6173ad01904f8dd21c
Cipher: aria-256-cfb | Key Length: 32 | IV Length: 16 | Key: c1053cf10540ac622e3258749d57b3a0ae11b39dec94f060f04e51cc29176829 | IV: d72e375301205c3c9bf43ab5c58de451
Cipher: aria-256-cfb1 | Key Length: 32 | IV Length: 16 | Key: 844df703ad707311a2c44666ec58557b5cbed42fc8fd8d9b612f0464905d882c | IV: 9cda1c8b53df66eafc2277603c959948
Cipher: aria-256-cfb8 | Key Length: 32 | IV Length: 16 | Key: 647b22d65dde849982f6f53f786b721745cbf84a32f2c60f203f0c3f2fb45af5 | IV: f1e92ec24e44d47993b4d3bf332e468f
Cipher: aria-256-ctr | Key Length: 32 | IV Length: 16 | Key: a4bbe5a9bc4d8188bb63d91d968cc52b6ffff0089d8a6b12c87ec5ced7a977b3 | IV: 7d930d12164d7f554bfd4d6dc5617002
Cipher: aria-256-ecb | Key Length: 32 | IV Length: NaN | Key: be3d8492d667a66c864dce1999b934cb60616bff804b20662ae188378133af88 | IV:
Cipher: aria-256-gcm | Key Length: 32 | IV Length: 12 | Key: 4d566d39c152689dbf3632d3c549b34cf9d6f1445dd7df0d634ebe64ea85b111 | IV: 230252ecbaf72d67d8d17ab7
Cipher: aria-256-ofb | Key Length: 32 | IV Length: 16 | Key: ec534c753151b4ed8047406ba22ce0d0fa1d824cd4d89f87f1d56362045c1b0d | IV: 0ca9d9f14f2307633ef1acc02c789285
Cipher: aria128 | Key Length: 16 | IV Length: 16 | Key: a8ef1df7cfa61bedfb733dd3647f05d6 | IV: 977f479040ded08d17c1f076feb823d3
Cipher: aria192 | Key Length: 24 | IV Length: 16 | Key: 89149e73c424bc488498c7b4c05ff9c2b8b653f578cac2f3 | IV: cad20166fadf00e9247d1c0aa46261d6
Cipher: aria256 | Key Length: 32 | IV Length: 16 | Key: 14bbd39bb29a5557abf6f081bbb6488f46eb92262c4397716a7c9094f37dd027 | IV: 38443ccb7f3327df097bc2ed28fc421c
Cipher: bf | Key Length: 16 | IV Length: 8 | Key: 11d0916167197fc6e1ec0d026b604ff3 | IV: 06f386fd2f5a8deb
Cipher: bf-cbc | Key Length: 16 | IV Length: 8 | Key: f93428fe3ccc68ff7b0599731eeea80e | IV: 201605249ed29db8
Cipher: bf-cfb | Key Length: 16 | IV Length: 8 | Key: 711cdaab7a353a8f54ea2b9173590262 | IV: d92ccde7c7799f89
Cipher: bf-ecb | Key Length: 16 | IV Length: NaN | Key: b00412224773eb2e686db1a69f1783b6 | IV:
Cipher: bf-ofb | Key Length: 16 | IV Length: 8 | Key: fcbeff0ea3e824d09d13ca024a11d4fe | IV: e46ff39e63ff7953
Cipher: blowfish | Key Length: 16 | IV Length: 8 | Key: 666e496a9f8e2ab16963f3e867fb8254 | IV: 9fff098aa82ef891
Cipher: camellia-128-cbc | Key Length: 16 | IV Length: 16 | Key: 55464b13aaa47c9d129e8a236637c622 | IV: f502b38e68cb696a45c9eac28fc927d3
Cipher: camellia-128-cfb | Key Length: 16 | IV Length: 16 | Key: 2b227715f2bfd27f1a25c2e4c84acd6a | IV: 6da6c51b3c90116e738f4cb2c431273a
Cipher: camellia-128-cfb1 | Key Length: 16 | IV Length: 16 | Key: ae3f34ca0094ab0aa0126db4d393b90a | IV: 11cb469a8175ee5f0390e054c8e67561
Cipher: camellia-128-cfb8 | Key Length: 16 | IV Length: 16 | Key: 512bdb6f40a4844b4a09b751eb59d403 | IV: b0680aa322b50237783374cbd02b17db
Cipher: camellia-128-ctr | Key Length: 16 | IV Length: 16 | Key: cf1e7cb391bded6cefda9b46bb5407a1 | IV: 16b821c14d908044568d5763859dbb7a
Cipher: camellia-128-ecb | Key Length: 16 | IV Length: NaN | Key: 4f437a6f88e9afbe913731ed110be763 | IV:
Cipher: camellia-128-ofb | Key Length: 16 | IV Length: 16 | Key: df5fa9424cf909d0be636a432f7573d7 | IV: 33e69fa42d68e8b15579da3922e50834
Cipher: camellia-192-cbc | Key Length: 24 | IV Length: 16 | Key: c1a0a51bf335ae96fad3c4a95f01410ce67711f67635f80e | IV: deb67895b92d2a9526fdff71ee14edde
Cipher: camellia-192-cfb | Key Length: 24 | IV Length: 16 | Key: ed729ff021c8ba1f02f89fc36a9f2b478d91c09dc646badc | IV: 39d7ac22012cdc188c5c41bf753b61aa
Cipher: camellia-192-cfb1 | Key Length: 24 | IV Length: 16 | Key: eeb90e1fa944606f284688e4f5ccf7b5b0b81b027274de56 | IV: 128f0a286fda1f90ed6a0a3767166a65
Cipher: camellia-192-cfb8 | Key Length: 24 | IV Length: 16 | Key: 01350c1d30b2d686d7f9f9ad6e0e24b5996f0b807eee4062 | IV: 38824e41cb8d24ee1ab939e42a7fe96e
Cipher: camellia-192-ctr | Key Length: 24 | IV Length: 16 | Key: 583e40fd11b8551436583f193595ac961e38b966dea3011e | IV: 9ea09769ae5b450f4a64d205131e4d29
Cipher: camellia-192-ecb | Key Length: 24 | IV Length: NaN | Key: 18dd7bf37bab366ab15a1aecbfec2208e00cb6eef62b5ac3 | IV:
Cipher: camellia-192-ofb | Key Length: 24 | IV Length: 16 | Key: 30cfa911702047b039997c22dced09435dfc0fda79726740 | IV: 698249b6d54914134c8924e821cdf2a1
Cipher: camellia-256-cbc | Key Length: 32 | IV Length: 16 | Key: ecd8f7d5ecbc959734d43334e8ae43b5bfcaba9294c9f19571a16b6ed13802f3 | IV: da4c2fd5753e08e0b64178eb7cf2c763
Cipher: camellia-256-cfb | Key Length: 32 | IV Length: 16 | Key: 71a87cc77f8f617a16651f7509a6cc7f76b11499adc93f2d70d953821eb8e236 | IV: 616bc5731cf5f0d5145db903bf4d9ce6
Cipher: camellia-256-cfb1 | Key Length: 32 | IV Length: 16 | Key: 37e7972de59bacb634f2767c903e6f09de212a4a44d755a8a0fb5837fbb1969f | IV: a18b8f57f02ee81b02ee62f389ba00df
Cipher: camellia-256-cfb8 | Key Length: 32 | IV Length: 16 | Key: 2b234d811e3c6583722cd27bb55f86b064a61b9656d9a3339c904aacae031657 | IV: 2b2b17edac6be65289ee6cd61f086961
Cipher: camellia-256-ctr | Key Length: 32 | IV Length: 16 | Key: 48aed9796b2d03ada412ed6a708bc745f16690cc5ce3a9ac70b43a131ee8c5ac | IV: e45dcf340d3b682b80254d6356917343
Cipher: camellia-256-ecb | Key Length: 32 | IV Length: NaN | Key: 520c6b34df8af86b97ebd29696bfe59e953ea2f22d36bdf1c6e2e0a4aadb242f | IV:
Cipher: camellia-256-ofb | Key Length: 32 | IV Length: 16 | Key: 2703dd1e80a8f1b34a56c5261c681a51819a55f8da425c1a4b81272e8409f6c7 | IV: 750536695ced6bb5f4975f3183f60ac7
Cipher: camellia128 | Key Length: 16 | IV Length: 16 | Key: dc9258d1b2a44ff8e487ad6b2c01621a | IV: 443e4c67d4f4f3b32e271dd00af26f50
Cipher: camellia192 | Key Length: 24 | IV Length: 16 | Key: 20cafac94b80821ea218562a91df0aad8df328641dd726a4 | IV: b96637b625feb859979283dde681e0d6
Cipher: camellia256 | Key Length: 32 | IV Length: 16 | Key: 09390a51f235ac46a1e76f8dcf942719e0825d28a24759a4fa33e59bcca0027c | IV: 63056f936ebd3dd91ff7ec193ca0b796
Cipher: cast | Key Length: 16 | IV Length: 8 | Key: fc9f575cc4a2be94853f779e1a316249 | IV: ecc1bee2fdf5f173
Cipher: cast-cbc | Key Length: 16 | IV Length: 8 | Key: 7e10fc0567a4d0a9669fc1f3c4a839c6 | IV: f91a967d22031e1b
Cipher: cast5-cbc | Key Length: 16 | IV Length: 8 | Key: 967bdfd03cad69ce324ebe958881eeb8 | IV: 97e5b0af3739de1e
Cipher: cast5-cfb | Key Length: 16 | IV Length: 8 | Key: a492d8085aff2b901c6db7d5efe7bf65 | IV: c1135ce62ca2384e
Cipher: cast5-ecb | Key Length: 16 | IV Length: NaN | Key: e981ee7932065eb0eff5c75a00594562 | IV:
Cipher: cast5-ofb | Key Length: 16 | IV Length: 8 | Key: 08eba5238b4ad8f13ebbc54f0a0eccab | IV: 5131f50a321d74f3
Cipher: chacha20 | Key Length: 32 | IV Length: 16 | Key: 5c9ca7d5f511c9b621259f323489eab77830f15f240b3bd8694d2522683e64c7 | IV: 18a034d72a1e4a84fa7e328c23e27315
Cipher: chacha20-poly1305 | Key Length: 32 | IV Length: 12 | Key: 3619a8cecf5da84e745c96ba126130420af5f6bb57a880db7b77ec01697f04d1 | IV: 22d34dac7c8ed8ff58f09185
Cipher: des | Key Length: 8 | IV Length: 8 | Key: ddc5fe41156890b1 | IV: 100b75e0b9715c67
Cipher: des-cbc | Key Length: 8 | IV Length: 8 | Key: 498a16ec32b1bd54 | IV: 0e05de948f759644
Cipher: des-cfb | Key Length: 8 | IV Length: 8 | Key: bca76f5322d344ec | IV: 127fba16236ae4dd
Cipher: des-cfb1 | Key Length: 8 | IV Length: 8 | Key: 6917352fb41c5a30 | IV: 28d2f18ca1496083
Cipher: des-cfb8 | Key Length: 8 | IV Length: 8 | Key: 38299a9886cf38f9 | IV: 8061265e5735d08e
Cipher: des-ecb | Key Length: 8 | IV Length: NaN | Key: 5f0a6fba5df95b0f | IV:
Cipher: des-ede | Key Length: 16 | IV Length: NaN | Key: 5b88a3ff3d4ff091e61c447d5d1dad9c | IV:
Cipher: des-ede-cbc | Key Length: 16 | IV Length: 8 | Key: fee921b348dd2974f2a425f523936f2c | IV: db2046fbdb21d7ad
Cipher: des-ede-cfb | Key Length: 16 | IV Length: 8 | Key: 29e90ffc4359c4b67a1c06a54a0ec213 | IV: 088151d927e86ff2
Cipher: des-ede-ecb | Key Length: 16 | IV Length: NaN | Key: f55e6048e65a9023018a6c71d3ceb848 | IV:
Cipher: des-ede-ofb | Key Length: 16 | IV Length: 8 | Key: 78ac6ed3216757dfcaf41aee9b747219 | IV: a4434ef084efbca2
Cipher: des-ede3 | Key Length: 24 | IV Length: NaN | Key: cd506e7479732900dd8d92256bf407ebee23d9b42b258581 | IV:
Cipher: des-ede3-cbc | Key Length: 24 | IV Length: 8 | Key: 43a657a3dc907c7781112511e992636469d8d21ceefdd0d7 | IV: 50e7c79ca0731622
Cipher: des-ede3-cfb | Key Length: 24 | IV Length: 8 | Key: e74a8f731453cccd9b202a947659f4f7267bde2e44668522 | IV: d664264771d33275
Cipher: des-ede3-cfb1 | Key Length: 24 | IV Length: 8 | Key: 5be085b4de41a18e7657eba00e5213696736efdbe6a2da23 | IV: ef54c09ead574bf7
Cipher: des-ede3-cfb8 | Key Length: 24 | IV Length: 8 | Key: eb7228b14a8299decbbb2c4a1d29cd9b8a2e2a78ad9a1ed8 | IV: 12aeeac49659c4a4
Cipher: des-ede3-ecb | Key Length: 24 | IV Length: NaN | Key: 5ce8321f4a25b13d9dc20232402abfa9e33de59de4b651b3 | IV:
Cipher: des-ede3-ofb | Key Length: 24 | IV Length: 8 | Key: bfca881a332943b29ab46dc1bfb7164b7726af177535fd9f | IV: 43b0c4b942a52148
Cipher: des-ofb | Key Length: 8 | IV Length: 8 | Key: fb2d3c426a7ce102 | IV: c459b53447829e60
Cipher: des3 | Key Length: 24 | IV Length: 8 | Key: 19d7a202a8cf9330c6b227097b18bf9f353cefe8ef7abc54 | IV: 825828498840bce3
Cipher: des3-wrap | Key Length: 24 | IV Length: NaN | Key: 3b14998c1071d0af29ded663d6c2fe23c899d4ee08c0a053 | IV:
Cipher: desx | Key Length: 24 | IV Length: 8 | Key: 275bc1ec32ebcc55d903c54bb304d2b164795bf40d263d7a | IV: c59135836adc9619
Cipher: desx-cbc | Key Length: 24 | IV Length: 8 | Key: 3ef452cd57d01d728a2a7410988ee1b63c8c85b16676ea1a | IV: 9e3b1fee607e6bbc
Cipher: id-aes128-CCM | Key Length: 16 | IV Length: 12 | Key: d4ac3525bce204908698c7ee579ae866 | IV: 8ccafe0230eac2f9953b6aad
Cipher: id-aes128-GCM | Key Length: 16 | IV Length: 12 | Key: c540b54dfa6f951998b02e870be2a380 | IV: 7758ccf99f45a405fe66f7aa
Cipher: id-aes128-wrap | Key Length: 16 | IV Length: 8 | Key: c6d65d407ee1172d13ccab6579111bf4 | IV: 6f74668abd1dd095
Cipher: id-aes128-wrap-pad | Key Length: 16 | IV Length: 4 | Key: 8ed3c6535049e067cb56db25f995fc66 | IV: 9f12312b
Cipher: id-aes192-CCM | Key Length: 24 | IV Length: 12 | Key: 7bcb76bbf331b840ce0bf2732044af547e738db7ce0297d5 | IV: 92e28ce3a1c9e8095bacd8c8
Cipher: id-aes192-GCM | Key Length: 24 | IV Length: 12 | Key: 04d4a383b951b6a5f086b25088a0cedd595fa7584032070f | IV: e92fbddde0b933dbdfd9ac49
Cipher: id-aes192-wrap | Key Length: 24 | IV Length: 8 | Key: e39eb0667f6e7b9f12b425d437cb239226ad4498a9f9745d | IV: 8c6dc3a95065d1aa
Cipher: id-aes192-wrap-pad | Key Length: 24 | IV Length: 4 | Key: c6675856a74d92ee4cab267d10330f05d85c5635fd3d095f | IV: f3104a7d
Cipher: id-aes256-CCM | Key Length: 32 | IV Length: 12 | Key: 1637ef0bde749038f82e5f2a7d66c62ed8c02cbe641c841f3b332f923ea7cb51 | IV: ccf7a9d4e46dcc5ec8a615fe
Cipher: id-aes256-GCM | Key Length: 32 | IV Length: 12 | Key: 380b3fc962e2c72962c8a248505cdc365988bc2d123c9a113fbbdc78737728ae | IV: df0fd2f45218410e08e74a86
Cipher: id-aes256-wrap | Key Length: 32 | IV Length: 8 | Key: cbd6a034424a40250cf8b38805b2471ca13d7c76fb21baee0de19d939167372a | IV: 01b22e04e67e1296
Cipher: id-aes256-wrap-pad | Key Length: 32 | IV Length: 4 | Key: aa5fcb632d80fe2d351699b5a716c5af1bb89eb7c1d3f500d540127ca2fb4172 | IV: 97b4b170
Cipher: id-smime-alg-CMS3DESwrap | Key Length: 24 | IV Length: NaN | Key: 0f01f3336e0110dd03318a38026f5b51dca2894027fbbd67 | IV:
Cipher: idea | Key Length: 16 | IV Length: 8 | Key: a740efebcbe419b7af97f1736df6a742 | IV: 323d2bcd14f8f8b7
Cipher: idea-cbc | Key Length: 16 | IV Length: 8 | Key: 13c8ada6e3291bc9ce077030ffaf0c33 | IV: 81fc2a9c87f916f3
Cipher: idea-cfb | Key Length: 16 | IV Length: 8 | Key: 6ec27e46a7480c2cd932a28d677756f0 | IV: 17fb9cc6b69002a4
Cipher: idea-ecb | Key Length: 16 | IV Length: NaN | Key: 50135f958cc72e4f0788bba995ba1ca2 | IV:
Cipher: idea-ofb | Key Length: 16 | IV Length: 8 | Key: 064debed1404db182501ceedf17b3acd | IV: 8e02fda39f948840
Cipher: rc2 | Key Length: 16 | IV Length: 8 | Key: 8d9e1605ec9c7823b07f3ab3bff7499f | IV: d680c7b5c4b223ee
Cipher: rc2-128 | Key Length: 16 | IV Length: 8 | Key: 9ae61b312bf910576bb2981ee6309af8 | IV: 7cf105510ca5b351
Cipher: rc2-40 | Key Length: 5 | IV Length: 8 | Key: c959240311 | IV: e49b55d7e6e02eca
Cipher: rc2-40-cbc | Key Length: 5 | IV Length: 8 | Key: 476a745e9a | IV: 8bd21b53d5cc9e21
Cipher: rc2-64 | Key Length: 8 | IV Length: 8 | Key: 55385cad5ff9ab8c | IV: 1cbb14876106ec6b
Cipher: rc2-64-cbc | Key Length: 8 | IV Length: 8 | Key: fb8e95d1fb168485 | IV: 117f0ab3c3b03012
Cipher: rc2-cbc | Key Length: 16 | IV Length: 8 | Key: 7e1b610ca28a83b69a610d1ad55b7f2e | IV: d733994513fb0aab
Cipher: rc2-cfb | Key Length: 16 | IV Length: 8 | Key: 134166c34e5c1aa0443c22c74cdd5bab | IV: 58890d49a72f87e1
Cipher: rc2-ecb | Key Length: 16 | IV Length: NaN | Key: a51c6a0c1312d8dbf99814572c375e9d | IV:
Cipher: rc2-ofb | Key Length: 16 | IV Length: 8 | Key: c3caaff9cc233e8ccf594ecc1924e38d | IV: d3ff34a6042fcef6
Cipher: rc4 | Key Length: 16 | IV Length: NaN | Key: 59e5a95abb2f6107775672a31c3821cb | IV:
Cipher: rc4-40 | Key Length: 5 | IV Length: NaN | Key: eb3762f3c0 | IV:
Cipher: rc4-hmac-md5 | Key Length: 16 | IV Length: NaN | Key: cee3ea81b823e643493ddb58f2093233 | IV:
Cipher: seed | Key Length: 16 | IV Length: 16 | Key: 7245ef1b3e7f6826ac01fb5d520d8d3d | IV: 74e173d47d75707383274581e474929a
Cipher: seed-cbc | Key Length: 16 | IV Length: 16 | Key: 26745d0439fb442199320330cb2b304a | IV: 1f7fcebc75ecebf68071927b10ab6f9d
Cipher: seed-cfb | Key Length: 16 | IV Length: 16 | Key: 6f20f662bbae18007b38cd4bb1a21bd0 | IV: 51fdba56353ec4f4bd022f216b61b6ce
Cipher: seed-ecb | Key Length: 16 | IV Length: NaN | Key: f2c806d76a266daf79c012188d6349d1 | IV:
Cipher: seed-ofb | Key Length: 16 | IV Length: 16 | Key: 705bb4907c5aba2f811664998357cd45 | IV: 2490c5d2c3b2f96a3dbd7d73e5dc6558
Cipher: sm4 | Key Length: 16 | IV Length: 16 | Key: ca453a9fee0cbc0ce33e7e9ead78c962 | IV: b3637b6bad631e24f83227ce8ad24156
Cipher: sm4-cbc | Key Length: 16 | IV Length: 16 | Key: ad031bd745a162e7b4aceee2e5c03f75 | IV: f43af4e547d166dd12099fa828a97dda
Cipher: sm4-cfb | Key Length: 16 | IV Length: 16 | Key: 4a1e17cc3c6a61818f9c91539aea9473 | IV: 99a4a87b05c4f6e486f4404465653ce9
Cipher: sm4-ctr | Key Length: 16 | IV Length: 16 | Key: 12cfc34b37541cff18c56bcd6ee10020 | IV: 8ea51b8552e3c3e1f213a70f265f4070
Cipher: sm4-ecb | Key Length: 16 | IV Length: NaN | Key: 0af340b2a3972ffcdb775eadc498d6f1 | IV:
Cipher: sm4-ofb | Key Length: 16 | IV Length: 16 | Key: 6c7749f1bd15b3cce0782c17ed136fd0 | IV: e8645b2c2f5f2ba47e776deec72cd364
Hope it helps.
Sources:
https://nodejs.org/api/crypto.html#crypto_crypto_pbkdf2sync_password_salt_iterations_keylen_digest