JWT Generation & Verification with HMAC SHA-256 Signature in .NET 6
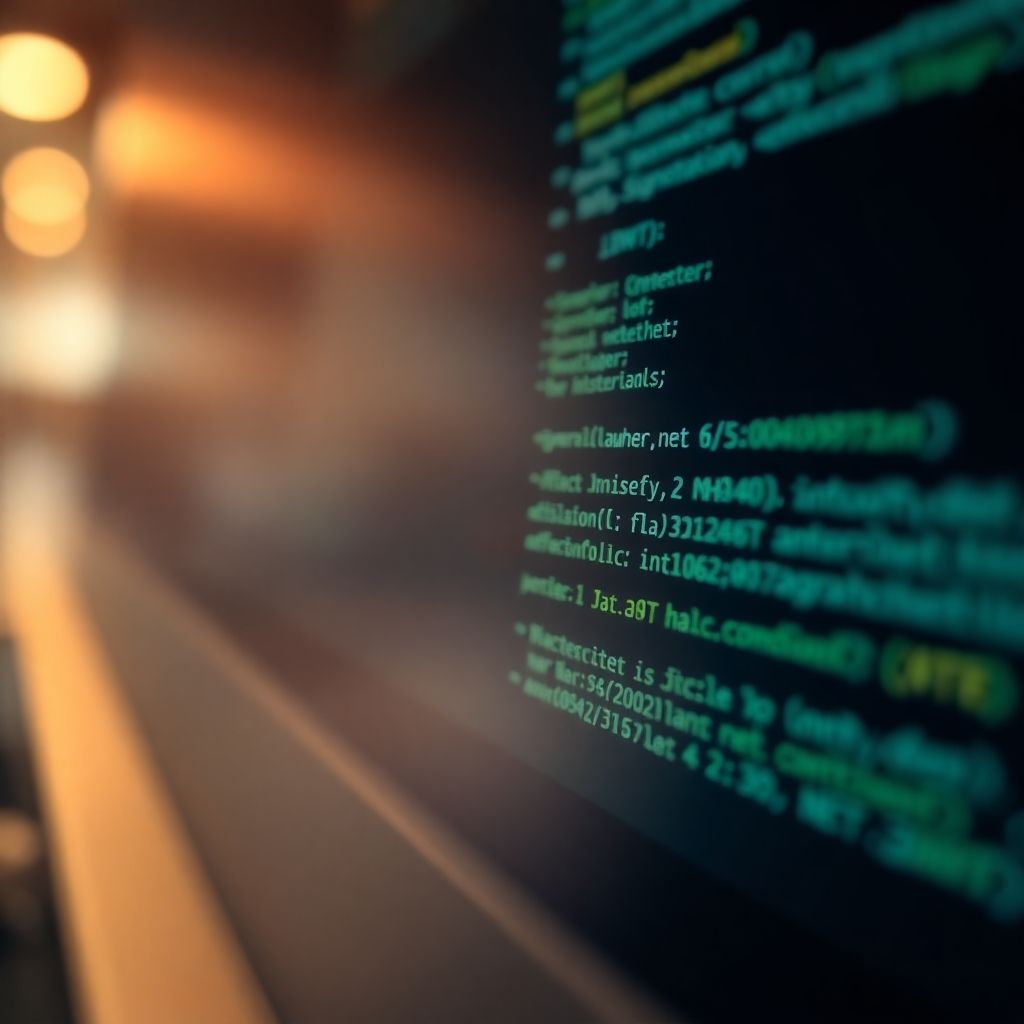
This code is the result of a chat session with AI using deepseek-coder-v2-latest model.
using System;
using System.IdentityModel.Tokens.Jwt;
using System.Security.Claims;
using System.Text;
using Microsoft.IdentityModel.Tokens;
class Program
{
static void Main(string[] args)
{
// Define the security key and algorithm to sign the token
var key = new SymmetricSecurityKey(Encoding.UTF8.GetBytes("your-256-bit-secret"));
var credentials = new SigningCredentials(key, SecurityAlgorithms.HmacSha256);
// Generate a JWT token (as shown in the previous example)
string jwt = GenerateJwtToken("your-issuer", "your-audience", key, credentials);
// Verify the signature of the generated JWT token
bool isValid = ValidateJwtSignature(jwt, "your-issuer", key);
// Write the result to the console
Console.WriteLine($"Is the JWT valid? {isValid}");
}
static string GenerateJwtToken(string issuer, string audience, SymmetricSecurityKey key, SigningCredentials credentials)
{
var claims = new[] {
new Claim(JwtRegisteredClaimNames.Sub, "user_id"),
new Claim(JwtRegisteredClaimNames.Name, "John Doe"),
new Claim(JwtRegisteredClaimNames.Email, "john.doe@example.com")
};
var token = new JwtSecurityToken(
issuer: issuer,
audience: audience,
claims: claims,
expires: DateTime.Now.AddMinutes(30),
signingCredentials: credentials
);
return new JwtSecurityTokenHandler().WriteToken(token);
}
static bool ValidateJwtSignature(string jwt, string issuer, SymmetricSecurityKey key)
{
try
{
var tokenHandler = new JwtSecurityTokenHandler();
var validationParameters = new TokenValidationParameters
{
ValidIssuer = issuer,
ValidAudience = issuer, // or the actual audience if different
ValidateIssuerSigningKey = true,
IssuerSigningKey = key,
ValidateLifetime = true,
ClockSkew = TimeSpan.Zero
};
SecurityToken validatedToken;
var principal = tokenHandler.ValidateToken(jwt, validationParameters, out validatedToken);
return true;
}
catch (Exception ex)
{
Console.WriteLine($"Validation failed: {ex.Message}");
return false;
}
}
}