How to create menu in Node.JS
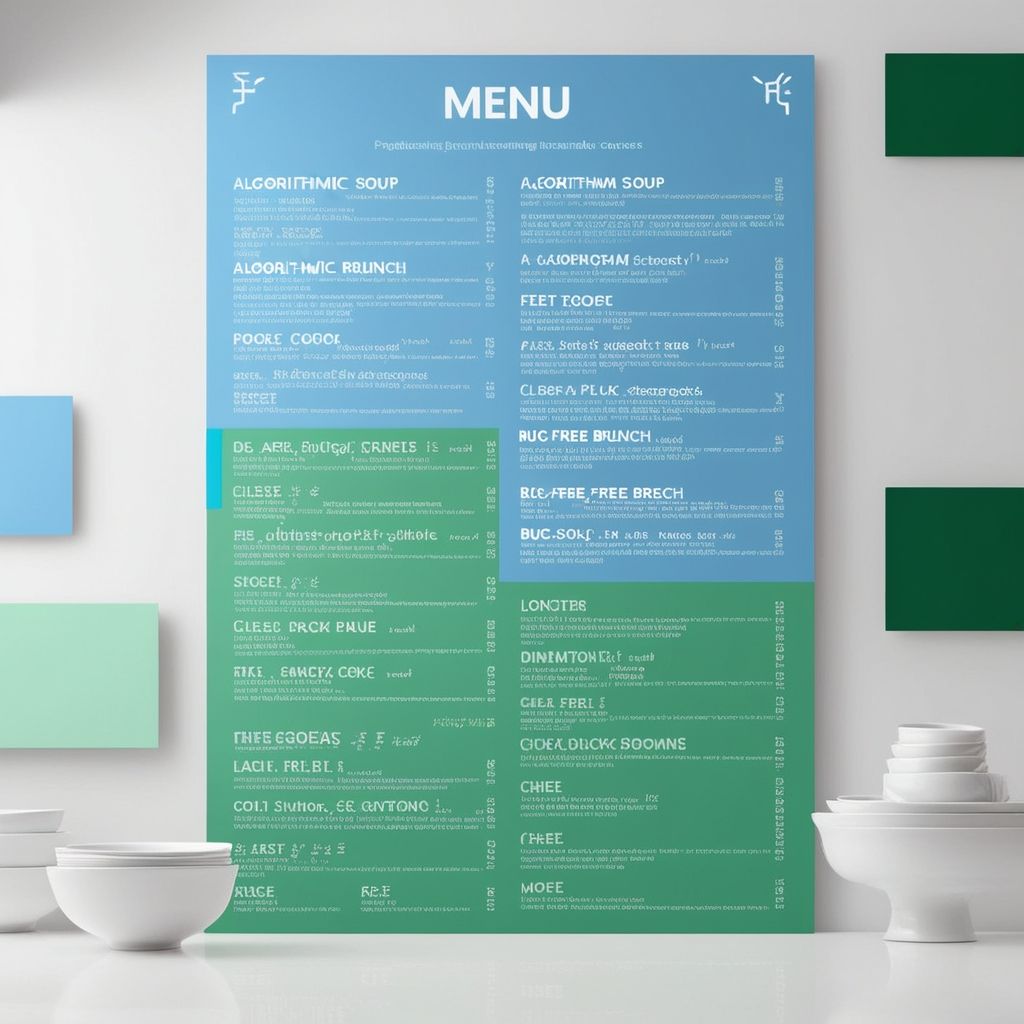
From the times of my Borland C programming where we had a fixed-width terminal that never changed size I had come up with the following code in Node.JS to now make it dynamically adjustable. The following code is just a skeleton and may be adjusted to your needs.
const readline = require('readline');
const rl = readline.createInterface({
input: process.stdin,
output: process.stdout,
});
function displayMenu() {
console.clear();
// Get console dimensions
const terminalWidth = process.stdout.columns; // Total width in characters
const terminalHeight = process.stdout.rows; // Total height in characters
// Calculate center position for menu text horizontally and vertically
const horizontalCenterOffset = (terminalWidth - 25) / 2; // Adjust '25' if your menu length changes
const verticalCenterOffset = (terminalHeight - 7) / 2; // Adjust '4' to account for the number of lines used by the menu
// Clear the console and print a centered title
console.log("\n".repeat(verticalCenterOffset)); // Move cursor down vertically
console.log(" ".repeat(horizontalCenterOffset) + "Select an option:".padEnd(terminalWidth));
console.log(" ".repeat(horizontalCenterOffset) + "1. Option 1");
console.log(" ".repeat(horizontalCenterOffset) + "2. Option 2");
console.log(" ".repeat(horizontalCenterOffset) + "3. Exit");
console.log();
rl.question(" ".repeat(horizontalCenterOffset) + "Enter your choice: ", (answer) => {
switch (parseInt(answer)) {
case 1:
console.clear();
console.log("You chose option 1!");
break;
case 2:
console.clear();
console.log("You chose option 2!");
break;
case 3:
console.clear();
console.log("Exiting...");
rl.close();
process.exit(0);
break;
default:
console.log("Invalid choice. Please enter a number between 1 and 3.");
}
// Wait for ENTER before redrawing the menu
rl.question('\nPress ENTER to continue...\n', () => {
main(); // Call main() again after ENTER
});
});
}
function main() {
displayMenu(); // Initially display the menu
}
main(); // Start the main function