Hash Strings in Python
Example program to understand how to hash strings with Python and run with python3.
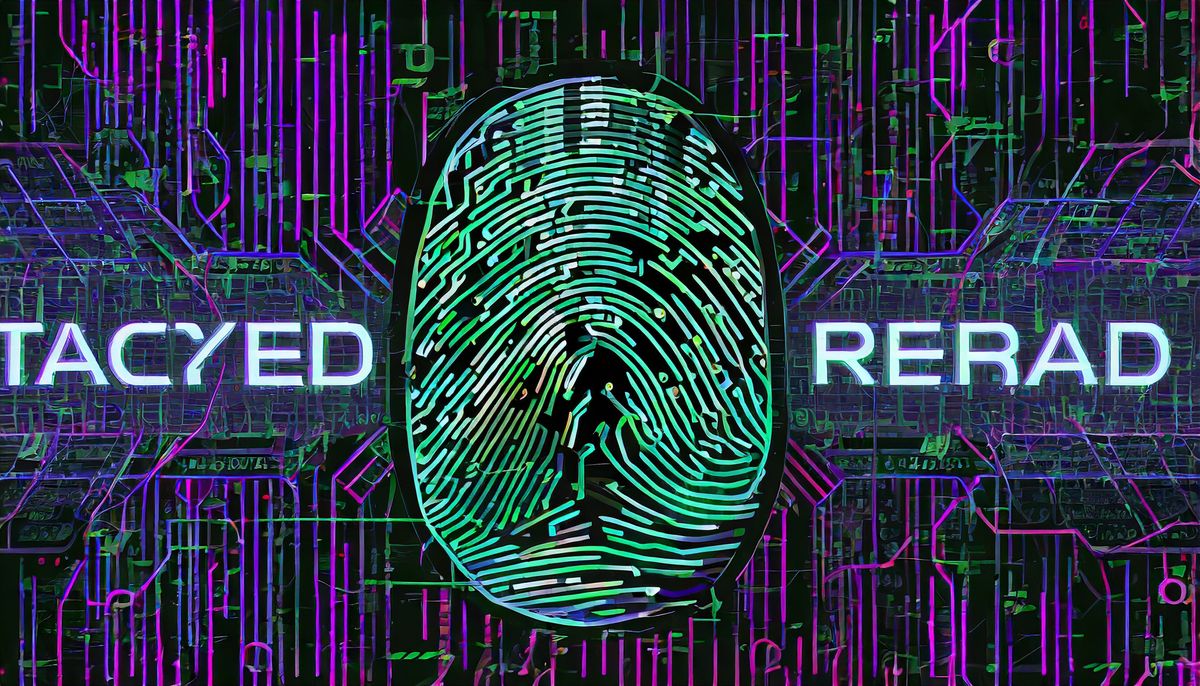
Here, is an example program to understand how it's done. Copy and paste this code into a file called hashStrings.py and run it with python3 hashStrings.py
.
import hashlib
textStr = 'Hello'
print('Text: ' + textStr)
print()
print('SHA-1 - ' + hashlib.sha1(textStr.encode('UTF-8')).hexdigest())
print('SHA-224 - ' + hashlib.sha224(textStr.encode('UTF-8')).hexdigest())
print('SHA-256 - ' + hashlib.sha256(textStr.encode('UTF-8')).hexdigest())
print('SHA-384 - ' + hashlib.sha384(textStr.encode('UTF-8')).hexdigest())
print('SHA-512 - ' + hashlib.sha512(textStr.encode('UTF-8')).hexdigest())
print('SHA-3-224 - ' + hashlib.sha3_224(textStr.encode('UTF-8')).hexdigest())
print('SHA-3-256 - ' + hashlib.sha3_256(textStr.encode('UTF-8')).hexdigest())
print('SHA-3-384 - ' + hashlib.sha3_384(textStr.encode('UTF-8')).hexdigest())
print('SHA-3-512 - ' + hashlib.sha3_512(textStr.encode('UTF-8')).hexdigest())
print('SHAKE-128 - ' + hashlib.shake_128(textStr.encode('UTF-8')).hexdigest(128))
print('SHAKE-256 - ' + hashlib.shake_256(textStr.encode('UTF-8')).hexdigest(256))
print('BLAKE-2B - ' + hashlib.blake2b(textStr.encode('UTF-8')).hexdigest())
print('BLAKE-2S - ' + hashlib.blake2s(textStr.encode('UTF-8')).hexdigest())
print('MD5 - ' + hashlib.md5(textStr.encode('UTF-8')).hexdigest())
Here's an example of the console output:
[terminator@skynet Hash_Strings]$ python3 hashStrings.py
Text: Hello
SHA-1 - f7ff9e8b7bb2e09b70935a5d785e0cc5d9d0abf0
SHA-224 - 4149da18aa8bfc2b1e382c6c26556d01a92c261b6436dad5e3be3fcc
SHA-256 - 185f8db32271fe25f561a6fc938b2e264306ec304eda518007d1764826381969
SHA-384 - 3519fe5ad2c596efe3e276a6f351b8fc0b03db861782490d45f7598ebd0ab5fd5520ed102f38c4a5ec834e98668035fc
SHA-512 - 3615f80c9d293ed7402687f94b22d58e529b8cc7916f8fac7fddf7fbd5af4cf777d3d795a7a00a16bf7e7f3fb9561ee9baae480da9fe7a18769e71886b03f315
SHA-3-224 - 4cf679344af02c2b89e4a902f939f4608bcac0fbf81511da13d7d9b9
SHA-3-256 - 8ca66ee6b2fe4bb928a8e3cd2f508de4119c0895f22e011117e22cf9b13de7ef
SHA-3-384 - df7e26e3d067579481501057c43aea61035c8ffdf12d9ae427ef4038ad7c13266a11c0a3896adef37ad1bc85a2b5bdac
SHA-3-512 - 0b8a44ac991e2b263e8623cfbeefc1cffe8c1c0de57b3e2bf1673b4f35e660e89abd18afb7ac93cf215eba36dd1af67698d6c9ca3fdaaf734ffc4bd5a8e34627
SHAKE-128 - 4131f8db5745776b48b86caa68d251fa9b19cf46b92b16289bb0c98e57e0e0de58c94a8b24ea4a9902a8307840b2ffbf2df2f0b1aa199ebea1c774e90c3b32a87702a95d054ae043fd33cbd312b01d9096771faaeb8a6ff5870752de3f1ac58c4267ecfe606f26d5bdaafa447fcc739c52bf4e8b87a5860904d7253162199c10
SHAKE-256 - 555796c90bfb8f3256a1cb0d7e574877fd48750e4147cf40aa43da122b4d64dafec00acf1ff59f4c3805f9589ec1ee3b712bc3701ce7d825cba56ca4942f6ffd648b34d7fe444f2838dadf1734c328b4e2f1d06ec0642d075f4ef0730aeafc45881532fdfd57837ce3bce0942da6aa8387469c785d86ace4d3be313c284130df23318e2d5c384bb024ee86b0b7049f912f324e9e254c4bd9f60a6fa1003291e454405d8c56b937c9460529a70d6b37f740660674b3e97c9385db07c10f054917f000c754645ad4402dbab8232ad97aaff584e3c24d5e331908189add625d62b07908e43d5468e8f103d35bc28226583adcb42961630370f08ec3b6d4451183c5
BLAKE-2B - ef15eaf92d5e335345a3e1d977bc7d8797c3d275717cc1b10af79c93cda01aeb2a0c59bc02e2bdf9380fd1b54eb9e1669026930ccc24bd49748e65f9a6b2ee68
BLAKE-2S - f73a5fbf881f89b814871f46e26ad3fa37cb2921c5e8561618639015b3ccbb71
MD5 - 8b1a9953c4611296a827abf8c47804d7
Sources: