Colors in console with Node.JS
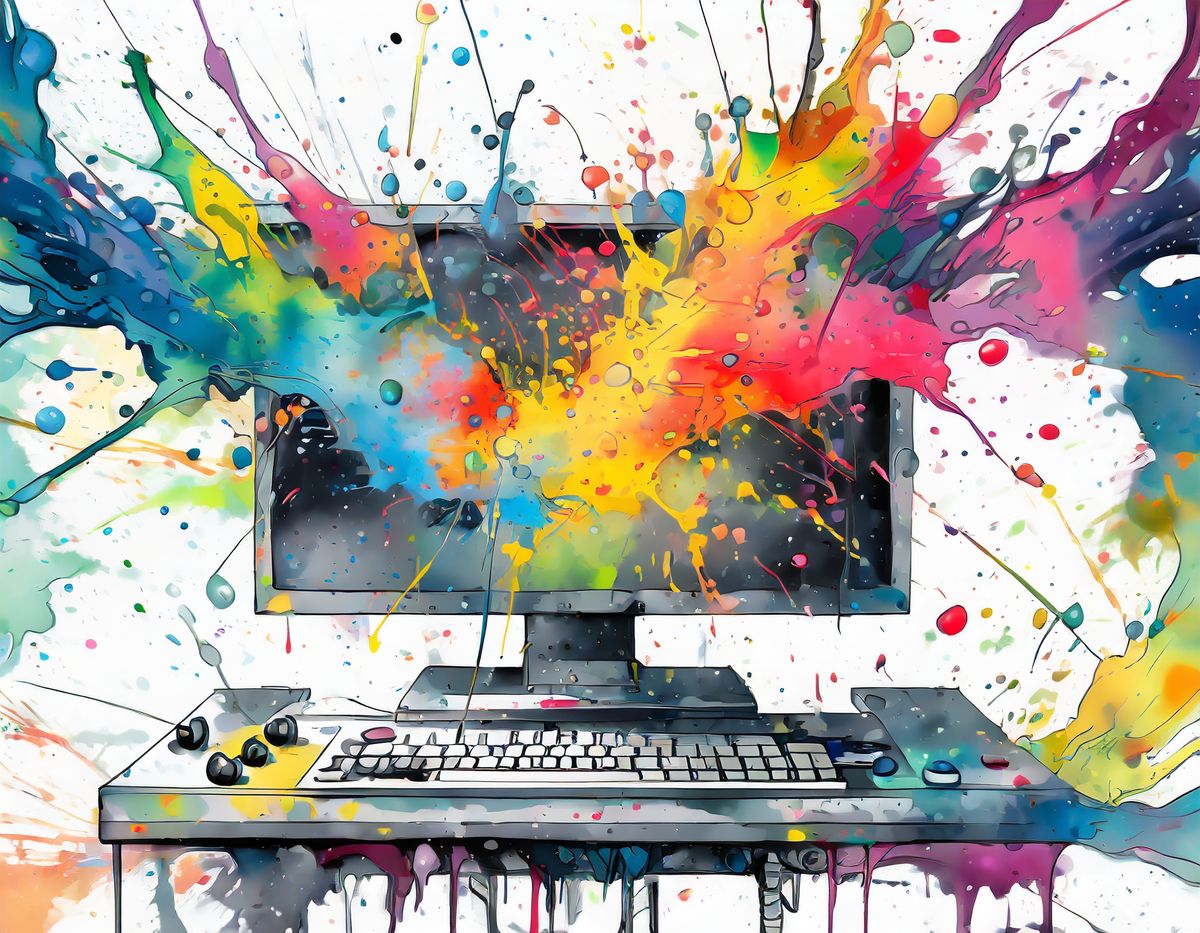
I recently wanted to make the output of my Node.JS applications a little more easy to read and I discovered that it's possible to print with some colors an other format. Here is a code that you can put inside a colors.js file and import it anywhere.
// Others ...
module.exports.RESET = "\x1b[0m";
module.exports.BOLD = "\x1b[1m";
module.exports.DIM = "\x1b[2m";
module.exports.UNDERSCORE = "\x1b[4m";
module.exports.BLINK = "\x1b[5m";
module.exports.REVERSE = "\x1b[7m";
module.exports.HIDDEN = "\x1b[8m";
// Foreground colors
module.exports.FG_BLACK = "\x1b[30m";
module.exports.FG_RED = "\x1b[31m";
module.exports.FG_GREEN = "\x1b[32m";
module.exports.FG_YELLOW = "\x1b[33m";
module.exports.FG_BLUE = "\x1b[34m";
module.exports.FG_MAGENTA = "\x1b[35m";
module.exports.FG_CYAN = "\x1b[36m";
module.exports.FG_WHITE = "\x1b[37m";
module.exports.FG_GRAY = "\x1b[90m";
// Background colors
module.exports.BG_BLACK = "\x1b[40m";
module.exports.BG_RED = "\x1b[41m";
module.exports.BG_GREEN = "\x1b[42m";
module.exports.BG_YELLOW = "\x1b[43m";
module.exports.BG_BLUE = "\x1b[44m";
module.exports.BG_MAGENTA = "\x1b[45m";
module.exports.BG_CYAN = "\x1b[46m";
module.exports.BG_WHITE = "\x1b[47m";
module.exports.BG_GRAY = "\x1b[100m";
Here is an example of how you can import it and use it in another file:
const fmt = require('colors');
console.log(fmt.RESET + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.BOLD + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.DIM + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.UNDERSCORE + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.BLINK + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.REVERSE + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.HIDDEN + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.FG_BLACK + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.FG_RED + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.FG_GREEN + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.FG_YELLOW + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.FG_BLUE + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.FG_MAGENTA + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.FG_CYAN + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.FG_WHITE + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.FG_GRAY + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.BG_BLACK + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.BG_RED + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.BG_GREEN + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.BG_YELLOW + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.BG_BLUE + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.BG_MAGENTA + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.BG_CYAN + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.BG_WHITE + "www.nodejslab.com" + fmt.RESET);
console.log(fmt.BG_GRAY + "www.nodejslab.com" + fmt.RESET);